Java Exception framework
A mechanism provided by the Java programming language to handle unexpected or exceptional situations that may occur during the execution of a program.
Exceptions represent situations where something goes wrong, and they allow you to gracefully respond to errors rather than letting them crash your program.
Checked exception example
import java.io.*;
public class W13aException1 {
public static void main(String[] args) {
System.out.println("java Exception example 1");
System.out.println("This will throw compile error");
File file = new File("E://file.txt");
FileReader fr = new FileReader(file);
}
}
Unchecked exception
With local error handling code.
public class W13bException2 {
public static void main(String[] args) {
System.out.println("java Exception example 2");
System.out.println("Throws runtime error");
int num[] = {1, 2, 3, 4};
int e = 3;
if (e < num.length) {
System.out.println(num[e]);
} else {
System.out.println("Index is bigger than the array size");
}
System.out.println("This code runs ONLY when there is NO error");
}
}
Exception handling version
You may try replacing ‘Exception’ with ‘ArrayIndexOutOfBoundsException’.
public class W13cException3 {
public static void main(String[] args) {
System.out.println("java Exception example 3");
System.out.println("Throws runtime error");
int num[] = {1, 2, 3, 4};
try {
int e = 5;
System.out.println(num[e]);
//ArrayIndexOutOfBoundsException
} catch (Exception ex) {
System.out.println("Index is bigger than the array size");
System.out.println(ex.getMessage());
System.out.println(ex);
}
System.out.println("This code runs ONLY when there is NO error");
}
}
Java Exception Hierarchy
Java has a hierarchy of exception classes. The base class is Throwable, and it has two main subclasses:
- Exception (for exceptional conditions that a program should catch) and
- Error (for severe errors that are not meant to be caught by regular programs).
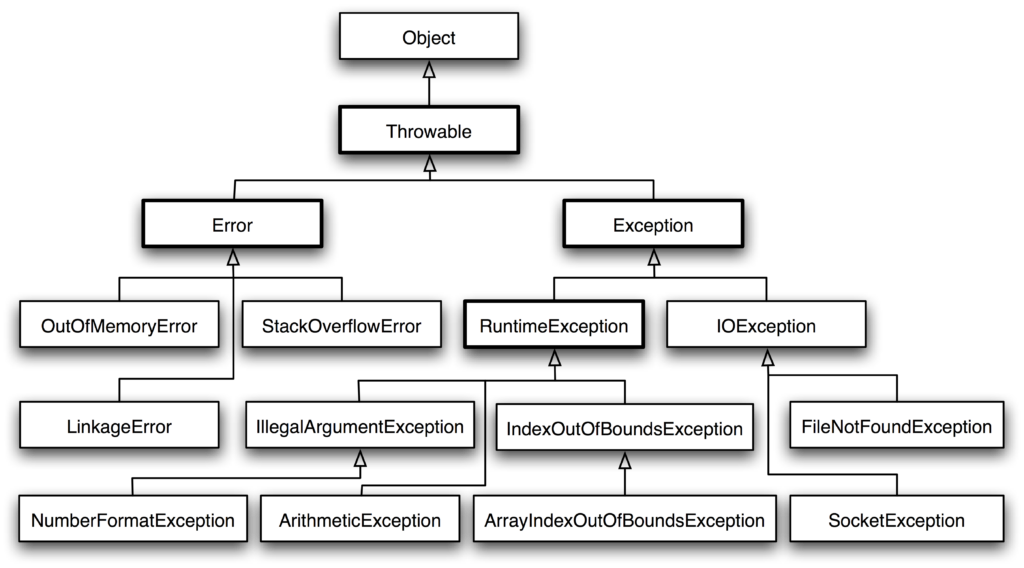
More info: https://rollbar.com/blog/java-list-of-all-checked-unchecked-exceptions/