We learn about the importance of “Generics” and “Collections”:
Generics in Java allow you to create classes, interfaces, and methods that operate on any type (or types) specified by the user. They provide a way to create reusable and type-safe code. We also talked about the mid-term exam/project and wrote the skeleton code to get started.
Generic class example
public class Box<T> {
private T content;
public void setContent(T content) {
this.content = content;
}
public T getContent() {
return content;
}
}
Tester
public class W12aGenerics2 {
public static void main(String[] args) {
Box<String> stringBox = new Box<>(); //create a Box object to store String type
stringBox.setContent("Hello, Generics!"); //store it
System.out.println("stringBox content = " + stringBox.getContent()); //retrieve it
Box<Integer> intBox = new Box<>(); //create a Box object to store Integer type
intBox.setContent(42);
System.out.println("intBox content = " + intBox.getContent());
}
}
Generic method example
public class W12bGenerics3 {
public static void displayInt(Integer[] m) {
System.out.println("From displayInt() ");
for (int one : m) {
System.out.println(one);
}
System.out.println("===============");
}
public static void displayDouble(Double[] t) {
System.out.println("From displayDouble() ");
for (double one : t) {
System.out.println(one);
}
System.out.println("===============");
}
public static void displayChar(Character[] c) {
System.out.println("From displayChar() ");
for (int one : c) {
System.out.println(one);
}
System.out.println("===============");
}
// generic method printArray
public static <T> void printArray(T[] inputArray) {
// Display array elements
for (T element : inputArray) {
System.out.printf("%s ", element);
}
System.out.println();
}
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
System.out.println("Display array content for different data types");
System.out.println("==============================================");
// Create arrays of Integer, Double and Character
Integer[] intArray = {1, 2, 3, 4, 5};
Double[] doubleArray = {1.1, 2.2, 3.3, 4.4};
Character[] charArray = {'H', 'E', 'L', 'L', 'O'};
//call different methods depending on the datatype
displayInt(intArray);
displayDouble(doubleArray);
displayChar(charArray);
//call the single generic array with any data type
printArray(intArray);
printArray(doubleArray);
printArray(charArray);
}
}
Java Collection Framework
The Java Collections Framework is a library of classes and interfaces that provide a unified architecture for representing and manipulating collections.
Collection example with List and ArrayList
import java.util.*;
/**
* ArrayList example
*
* A class that implements the List interface,
* providing functionality to store and manipulate an ordered collection of elements.
*
* @author azmeer
*/
public class W12cList {
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
List<String> names = new ArrayList<>();
names.add("Nimal");
names.add("Anil");
names.add("Mahesh");
System.out.println("No of elements: " + names.size());
System.out.println("Names (unsorted): " + names);
Collections.sort(names);
System.out.println("Names (sorted): " + names);
Collections.reverse(names);
System.out.println("Names (reverse sorted): " + names);
}
}
Here, we use ArrayList as the class that implements the List interface, providing functionality to store and manipulate an ordered collection of elements. Notice how easy it is to sort List items.
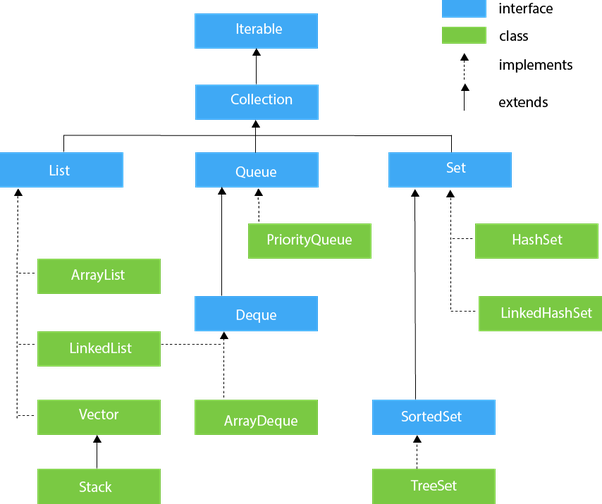