Advance Dart programming. We learned about Future, REST API access and other best practices.
Console user input
In Dart, you can get user input from the console using the stdin library, which is part of the dart:io library. Here’s a simple example of how you can get user input. Run it from the console (NOT by VSCode “run” command, which gives errors).
Keep in mind that this approach is suitable for console-based Dart applications. For Flutter applications or web-based Dart applications, you would typically use different methods to capture user input based on the platform and user interface components.
import 'dart:io';
void main() {
// Prompt the user for input
stdout.write('Enter your name: ');
// Read the user input from the console
var userName = stdin.readLineSync();
// Print a message using the user input
print('Hello, $userName! How are you doing today?');
}
null and null safe coding
In Dart, null is a special value that represents the absence of a value. It is often used to indicate that a variable or expression does not have a meaningful result or does not refer to any object.
void main() {
String nonNullableString = 'Hello'; // Non-nullable
nonNullableString = null; //throws an error
String? nullableString = null; // Nullable
}
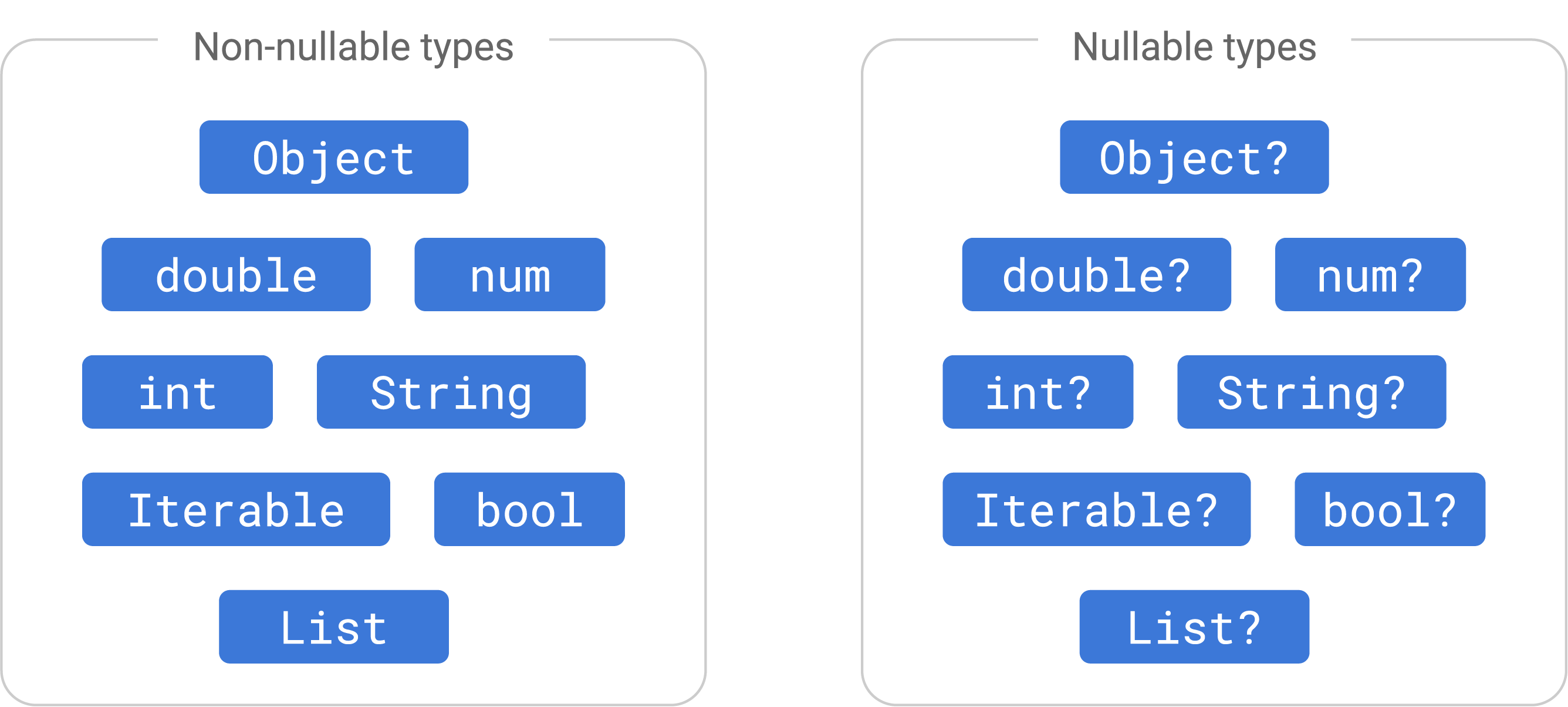
More on NULL safe coding
Correction
In the video recording I mentioned about Dart being multi-threaded. It is a mistake. The truth is Dart is similar to JavaScript when it comes to async programming.
Dart’s async and await keywords are used to work with asynchronous code, making it more readable and easier to reason about. Let’s break down Dart’s asynchronous programming model in a way that’s easy to understand.
What is Asynchronous Programming?
In programming, tasks are often performed one after the other, in a sequence. This is called synchronous programming. Asynchronous programming allows us to start a task, then move on to do other things while waiting for the first task to finish.
Futures: Representing Future Values
In Dart, we use something called a Future to represent a value (or an error) that will be available at some time in the future. It’s like telling Dart, “Hey, I’m going to do something that might take a while. Here’s a ‘future’ value that you can use when I’m done.”
Asynchronous Functions with async and await:
When we have a function that performs an asynchronous operation, we mark that function with the async keyword. We use the await keyword to tell Dart to wait for the completion of an asynchronous operation before moving on.
Example: Waiting for a Delayed Task:
//main code: bin/future_test.dart
import 'package:future_test/fetch_data.dart' as future_test;
void main(List<String> arguments) {
print('Testing Dart async/await system.');
future_test.fetchData().then(
(value) =>
print(value), // Called when the Future completes successfully
onError: (error) =>
print("Error: $error"), // Called when there's an error
);
print("This line executes immediately");
}
//library function: /lib/fetch_data.dart
Future<String> fetchData() {
// Simulating an asynchronous operation
return Future.delayed(
Duration(seconds: 5), () => 'Data fetched after 5 seconds successfully');
}
Here, Future.delayed simulates a task that takes 1 second to complete. The await keyword makes sure that the program waits for this task to finish before moving on to the next line.
Event Loop: The Brain Behind Asynchrony:
Dart has something called an “event loop” that keeps track of all the tasks that are running. It checks if a task is done, and if so, it executes the code associated with that task.
Why Use Asynchronous Programming in Dart?
- Efficiency: While waiting for one task to finish, Dart can start working on other tasks, making our programs more efficient.
- Responsive UI: In apps, we can keep the user interface responsive even when performing time-consuming tasks.
Remember: It’s Like Cooking:
Imagine you’re cooking. You put something on the stove to simmer. Instead of staring at the pot until it’s done, you can start chopping vegetables or setting the table. Similarly, in Dart, we can start tasks, then work on other things while waiting for the initial tasks to complete.
Dart’s asynchronous model is like having a personal assistant that can manage multiple tasks for you, allowing your program to do more things at the same time without getting stuck.